Serialization Example
Note that you need to add a reference to System.Runtime.Serialization.Formatters.Soap.dll to your project before compiling the code below. Unlike the BinaryFormatter class, the SoapFormatter class is not included by default.
using System;
using System.IO;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Formatters.Binary;
using System.Runtime.Serialization.Formatters.Soap;
namespace SerializationExample
{
[Serializable]
class ObjectToSerialize
{
string sName;
int iCount;
double dPrice;
public ObjectToSerialize(string newName, int newCount, double newPrice)
{
sName = newName;
iCount = newCount;
dPrice = newPrice;
}
}
class Program
{
static void Main(string[] args)
{
ObjectToSerialize o = new ObjectToSerialize("Video Game", 1, 50.00);
// Create the files to save the data to
FileStream binary_fs = new FileStream("Serialization_Example.bin", FileMode.Create);
FileStream xml_fs = new FileStream("Serialization_Example.xml", FileMode.Create);
// Create a BinaryFormatter object to perform the serialization
BinaryFormatter bf = new BinaryFormatter();
// Create a SoapFormatter object to perform serialization
SoapFormatter sf = new SoapFormatter();
// Use the BinaryFormatter object to serialize the data to the file
bf.Serialize(binary_fs, o);
// Use the SoapFormatter object to serialize the data to the file
sf.Serialize(xml_fs, o);
// Close the files
binary_fs.Close();
xml_fs.Close();
}
}
}
Binary Results
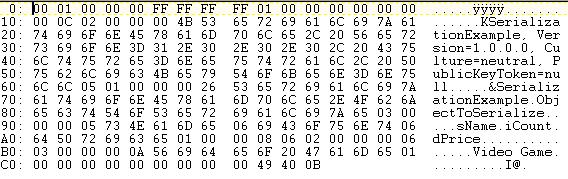
The XML results of the SOAP Serialization can be found here: SOAP Serialization Results
Additional Resources
BinaryFormatter Class (Microsoft)
SoapFormatter Class (Microsoft)
No comments:
Post a Comment